Federico Ramallo
Aug 3, 2024
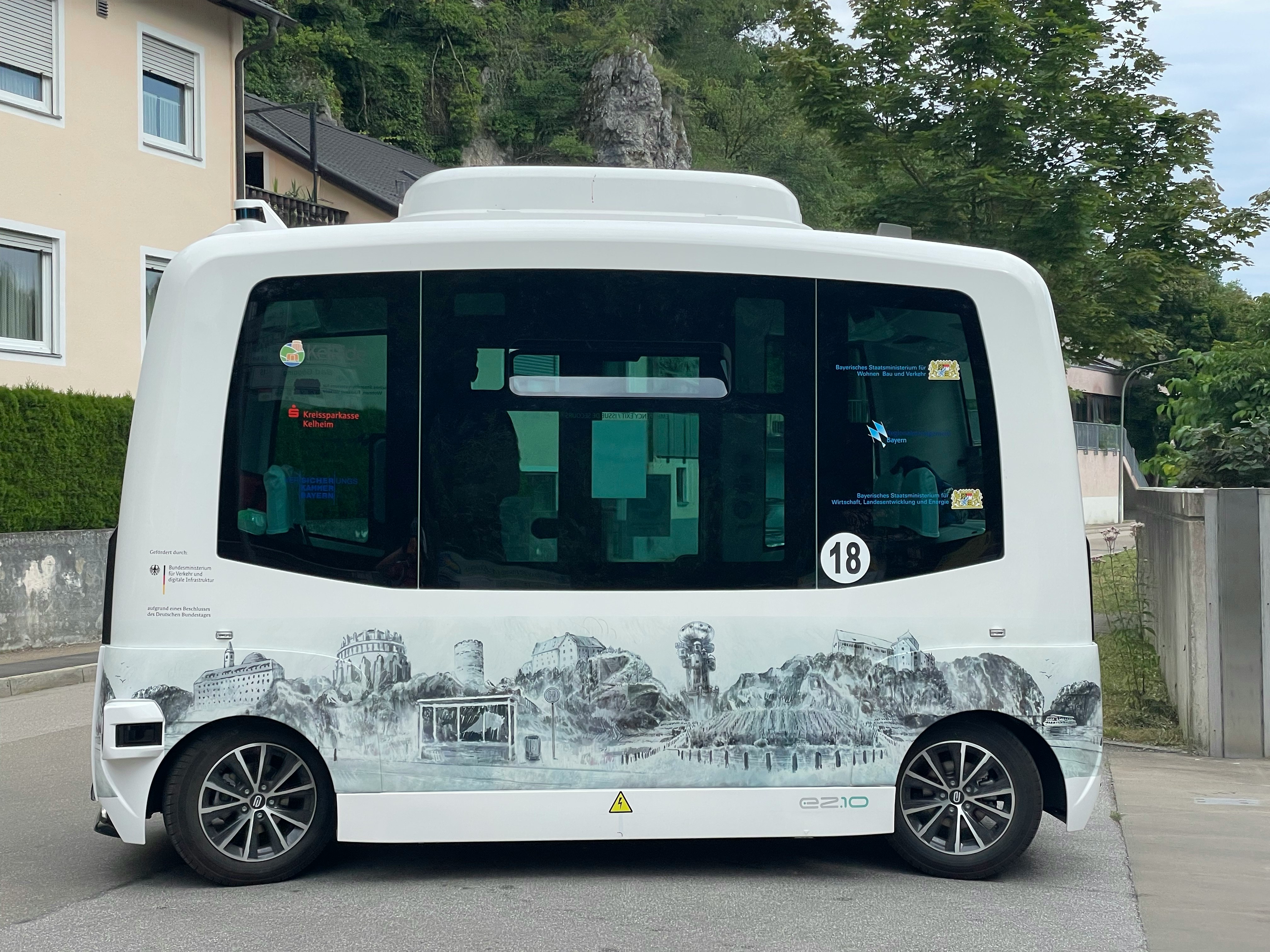
Automate Your RSpec Tests with GitHub Actions. Here’s How.
Federico Ramallo
Aug 3, 2024
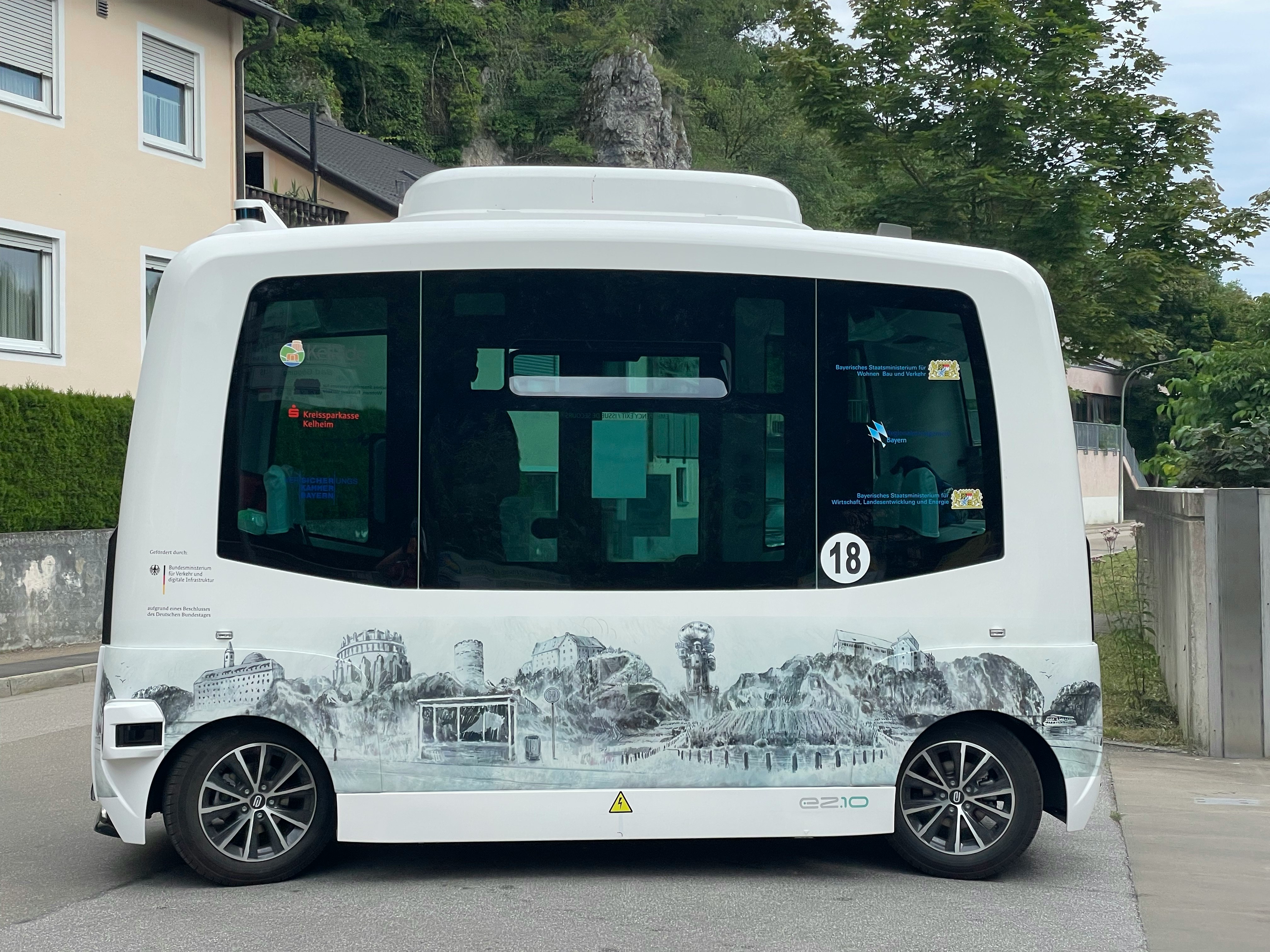
Automate Your RSpec Tests with GitHub Actions. Here’s How.
Federico Ramallo
Aug 3, 2024
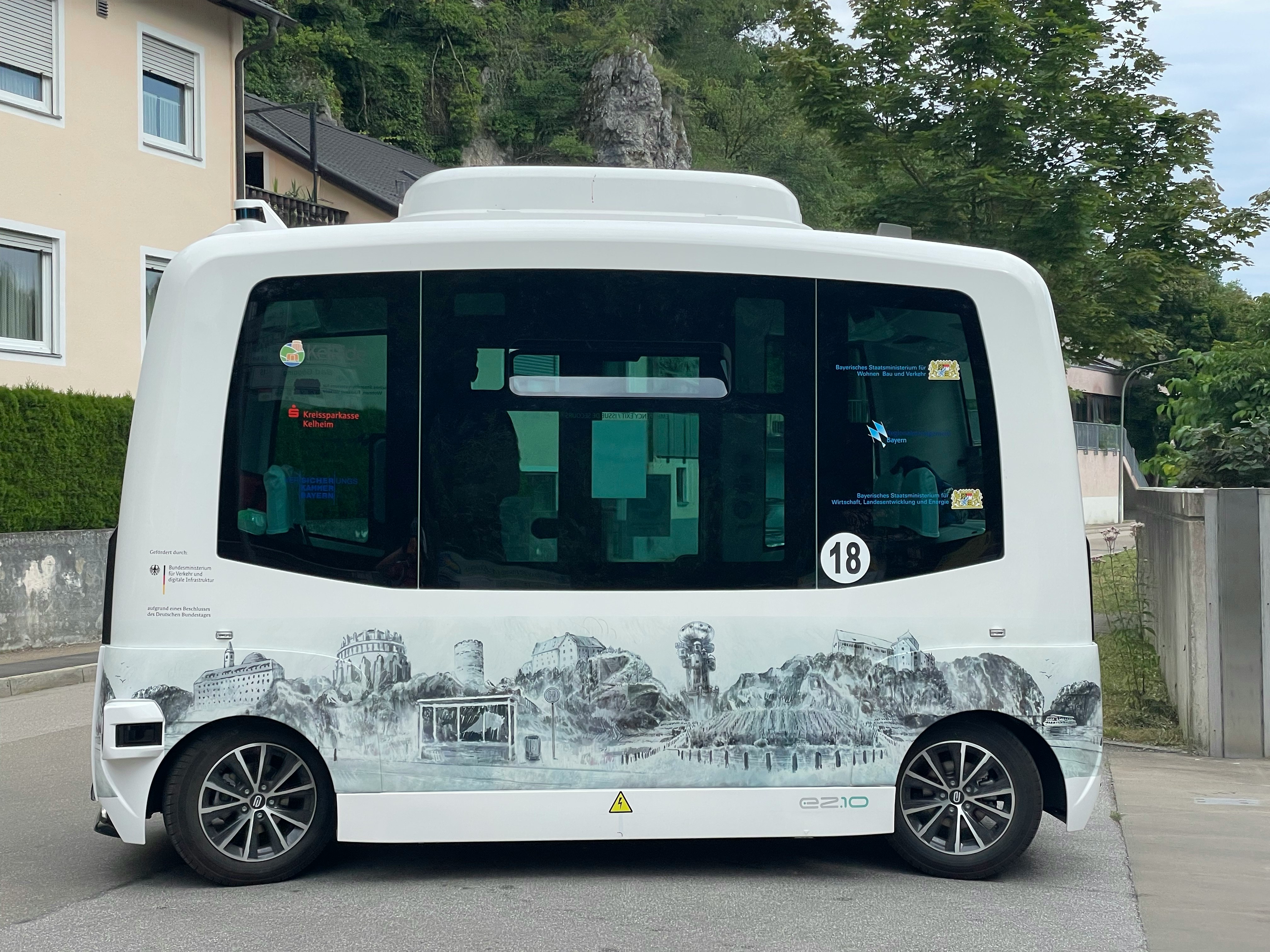
Automate Your RSpec Tests with GitHub Actions. Here’s How.
Federico Ramallo
Aug 3, 2024
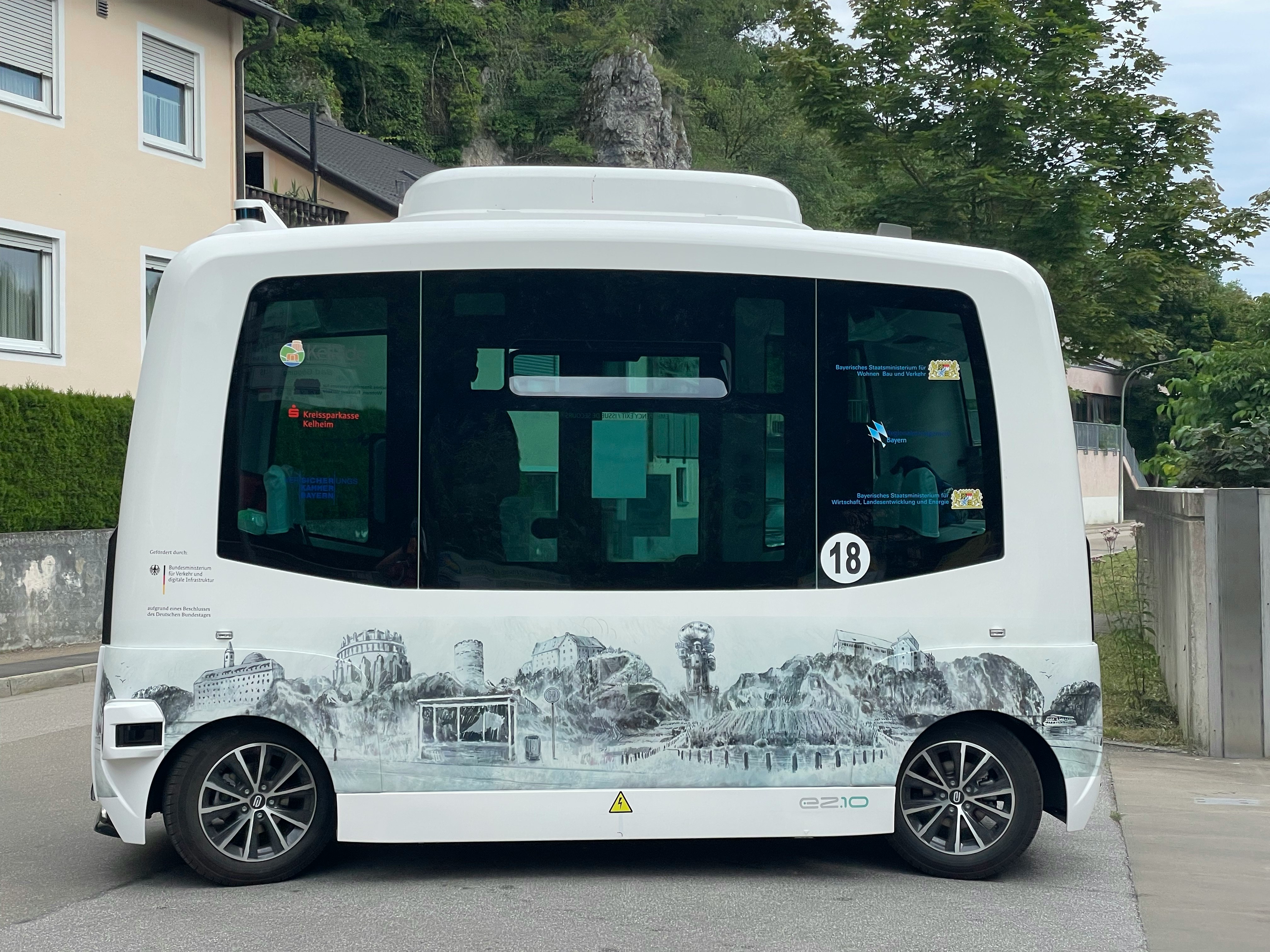
Automate Your RSpec Tests with GitHub Actions. Here’s How.
Federico Ramallo
Aug 3, 2024
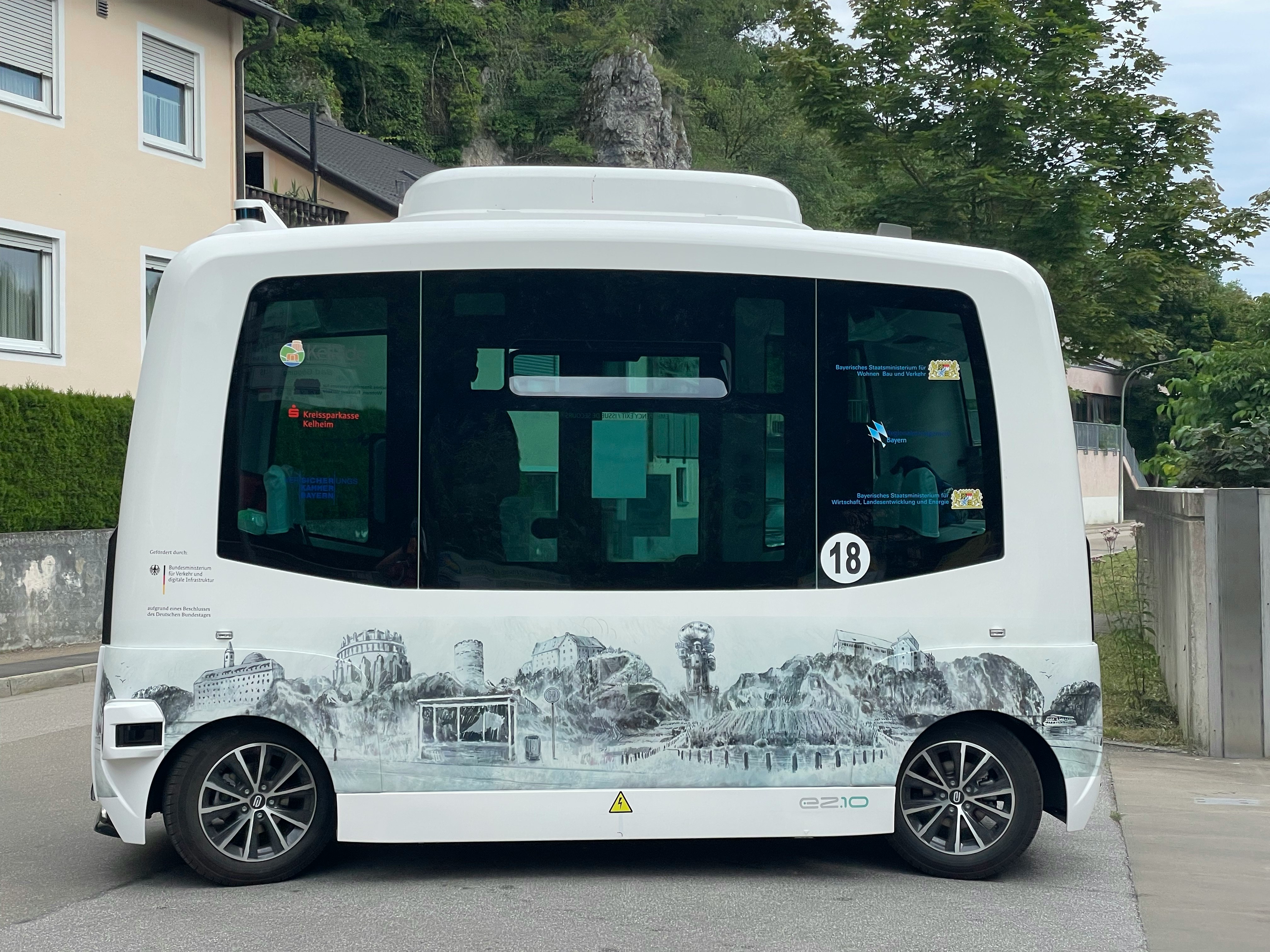
Automate Your RSpec Tests with GitHub Actions. Here’s How.
GitHub Actions is a feature that enables developers to automate their software workflows. With 2000 free minutes per month, developers can build, test, and deploy code automatically, integrating this functionality directly into their GitHub repositories. The automation includes critical processes like unit testing and deployment, which helps streamline continuous integration and continuous delivery (CI/CD) efforts.
GitHub Actions is particularly useful in ensuring that code remains functional and bug-free by running tests on every push or pull request. This saves developers time by allowing them to focus on writing code without worrying about manual deployment and testing.
To set up GitHub Actions for automated RSpec testing, the process starts by creating a folder structure within the repository: .github/workflows. Within this structure, a YAML file defines the specific actions that will run. The YAML file specifies when the actions should be triggered, typically on push or pull request events, and identifies the folder containing the code to be tested.
In the YAML configuration, developers define the operating environment for the tests, usually specifying the latest version of Ubuntu. The environment includes services like PostgreSQL, Redis, and Elasticsearch to mimic the actual production setup. These services are essential for ensuring the tests run accurately, particularly when dealing with databases and other back-end systems.
The YAML file includes commands to install dependencies like Ruby and PostgreSQL. Ruby for Rails projects, and PostgreSQL is necessary for running the database used in testing. After setting up these dependencies, the YAML script installs the necessary Ruby gems through bundle install. This ensures that all the libraries and packages needed for the project are available in the testing environment.
Once the environment is prepared, the script sets up the database by creating it and loading the schema. This step aligns the test environment's database structure with the codebase, ensuring that the tests run against a fully functional database setup.
The final step in the process is running the RSpec suite. RSpec is a popular testing framework for Ruby that allows developers to write tests that validate the correctness of their code. By running the tests automatically on every code push, developers can ensure that new changes do not break existing functionality. This automatic testing saves time and effort by catching bugs early in the development process, reducing the risk of errors making it to production.
One of the key advantages of this setup is that it eliminates the need for manual testing or deployment. The tests run automatically in the background whenever code is pushed to the repository. Developers can work with the assurance that their code is being tested, and once all tests pass, the code is ready for deployment. This process simplifies the workflow and ensures a more reliable, automated approach to testing and deployment.
GitHub Actions provides a free machine for this automation, significantly reducing infrastructure costs for teams. The machine is only activated when code is pushed or a pull request is created, allowing the team to use resources efficiently. Once the tests are completed, the machine can shut down, saving valuable computing time and resources.
Overall, automating testing with GitHub Actions enhances productivity and reduces errors in the development process. By setting up a continuous testing system with RSpec and integrating it into GitHub, developers can streamline their workflows, allowing them to focus on writing quality code. This approach ensures that the codebase remains stable, and developers can deploy updates with confidence, knowing that their tests will catch any potential issues early.
So this is how my github action looks like:
# .github/workflows/rails-tests.yml
env:
DB_NAME: talent_test
DB_USERNAME: postgres
DB_PASSWORD: postgres
DB_HOST: localhost
POSTGRES_HOST_AUTH_METHOD: trust
RAILS_ENV: test
name: Rails Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
strategy:
fail-fast: false
matrix:
include:
# - name: traceroute
# command: bin/rails traceroute
# requires_gems: true
# requires_db: false
# - name: brakeman
# command: bundle exec brakeman
# requires_gems: true
# requires_db: false
# - name: database_consistency
# command: bundle exec database_consistency
# requires_gems: true
# requires_db: true
# - name: erblint
# command: bundle exec erblint --lint-all
# requires_gems: true
# requires_db: false
- name: rspec_controllers
command: bundle exec rspec spec/controllers
requires_gems: true
requires_db: true
- name: rspec_helpers
command: bundle exec rspec spec/helpers spec/helpers
requires_gems: true
requires_db: true
- name: rspec_models
command: bundle exec rspec spec/mailers spec/models
requires_gems: true
requires_db: true
# - name: rspec_requests
# command: bundle exec rspec spec/requests
# requires_gems: true
# requires_db: true
# - name: rubocop
# command: bundle exec rubocop --parallel
# requires_gems: true
# requires_db: false
# - name: eslint
# command: node_modules/.bin/eslint --ext .js --ext .jsx --ext .json app/javascript
# requires_gems: false
# requires_db: false
# - name: gherkin-lint
# command: node_modules/.bin/gherkin-lint spec/acceptance/features
# requires_gems: false
# requires_db: false
# - name: jest
# command: node_modules/.bin/jest --ci --json --coverage
# requires_gems: false
# requires_db: false
# - name: stylelint
# command: node_modules/.bin/stylelint "app/**/*.scss"
# requires_gems: false
# requires_db: false
name: ${{ matrix.name }}
services:
postgres:
image: postgres:11.6
env:
POSTGRES_USER: ${{ env.DB_USERNAME }}
POSTGRES_DB: ${{ env.DB_NAME }}
POSTGRES_PASSWORD: ${{ env.DB_PASSWORD }}
POSTGRES_HOST_AUTH_METHOD: ${{ env.POSTGRES_HOST_AUTH_METHOD }}
ports:
- 5432:5432
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Setup Ruby
uses: ruby/setup-ruby@v1
if: ${{ matrix.requires_gems }}
- name: Ruby gem cache
uses: actions/cache@v2
with:
path: vendor/bundle
key: ${{ runner.os }}-gems-${{ hashFiles('**/Gemfile.lock') }}
restore-keys: ${{ runner.os }}-gems-
if: ${{ matrix.requires_gems }}
- name: Install gems
run: |
bundle config path vendor/bundle
bundle install --jobs 4 --retry 3 --without production
if: ${{ matrix.requires_gems }}
- name: Setup test database
run: bin/rails db:create db:migrate
if: ${{ matrix.requires_db }}
- name: Run ${{ matrix.name }}
run: ${{ matrix.command }}
GitHub Actions is a feature that enables developers to automate their software workflows. With 2000 free minutes per month, developers can build, test, and deploy code automatically, integrating this functionality directly into their GitHub repositories. The automation includes critical processes like unit testing and deployment, which helps streamline continuous integration and continuous delivery (CI/CD) efforts.
GitHub Actions is particularly useful in ensuring that code remains functional and bug-free by running tests on every push or pull request. This saves developers time by allowing them to focus on writing code without worrying about manual deployment and testing.
To set up GitHub Actions for automated RSpec testing, the process starts by creating a folder structure within the repository: .github/workflows. Within this structure, a YAML file defines the specific actions that will run. The YAML file specifies when the actions should be triggered, typically on push or pull request events, and identifies the folder containing the code to be tested.
In the YAML configuration, developers define the operating environment for the tests, usually specifying the latest version of Ubuntu. The environment includes services like PostgreSQL, Redis, and Elasticsearch to mimic the actual production setup. These services are essential for ensuring the tests run accurately, particularly when dealing with databases and other back-end systems.
The YAML file includes commands to install dependencies like Ruby and PostgreSQL. Ruby for Rails projects, and PostgreSQL is necessary for running the database used in testing. After setting up these dependencies, the YAML script installs the necessary Ruby gems through bundle install. This ensures that all the libraries and packages needed for the project are available in the testing environment.
Once the environment is prepared, the script sets up the database by creating it and loading the schema. This step aligns the test environment's database structure with the codebase, ensuring that the tests run against a fully functional database setup.
The final step in the process is running the RSpec suite. RSpec is a popular testing framework for Ruby that allows developers to write tests that validate the correctness of their code. By running the tests automatically on every code push, developers can ensure that new changes do not break existing functionality. This automatic testing saves time and effort by catching bugs early in the development process, reducing the risk of errors making it to production.
One of the key advantages of this setup is that it eliminates the need for manual testing or deployment. The tests run automatically in the background whenever code is pushed to the repository. Developers can work with the assurance that their code is being tested, and once all tests pass, the code is ready for deployment. This process simplifies the workflow and ensures a more reliable, automated approach to testing and deployment.
GitHub Actions provides a free machine for this automation, significantly reducing infrastructure costs for teams. The machine is only activated when code is pushed or a pull request is created, allowing the team to use resources efficiently. Once the tests are completed, the machine can shut down, saving valuable computing time and resources.
Overall, automating testing with GitHub Actions enhances productivity and reduces errors in the development process. By setting up a continuous testing system with RSpec and integrating it into GitHub, developers can streamline their workflows, allowing them to focus on writing quality code. This approach ensures that the codebase remains stable, and developers can deploy updates with confidence, knowing that their tests will catch any potential issues early.
So this is how my github action looks like:
# .github/workflows/rails-tests.yml
env:
DB_NAME: talent_test
DB_USERNAME: postgres
DB_PASSWORD: postgres
DB_HOST: localhost
POSTGRES_HOST_AUTH_METHOD: trust
RAILS_ENV: test
name: Rails Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
strategy:
fail-fast: false
matrix:
include:
# - name: traceroute
# command: bin/rails traceroute
# requires_gems: true
# requires_db: false
# - name: brakeman
# command: bundle exec brakeman
# requires_gems: true
# requires_db: false
# - name: database_consistency
# command: bundle exec database_consistency
# requires_gems: true
# requires_db: true
# - name: erblint
# command: bundle exec erblint --lint-all
# requires_gems: true
# requires_db: false
- name: rspec_controllers
command: bundle exec rspec spec/controllers
requires_gems: true
requires_db: true
- name: rspec_helpers
command: bundle exec rspec spec/helpers spec/helpers
requires_gems: true
requires_db: true
- name: rspec_models
command: bundle exec rspec spec/mailers spec/models
requires_gems: true
requires_db: true
# - name: rspec_requests
# command: bundle exec rspec spec/requests
# requires_gems: true
# requires_db: true
# - name: rubocop
# command: bundle exec rubocop --parallel
# requires_gems: true
# requires_db: false
# - name: eslint
# command: node_modules/.bin/eslint --ext .js --ext .jsx --ext .json app/javascript
# requires_gems: false
# requires_db: false
# - name: gherkin-lint
# command: node_modules/.bin/gherkin-lint spec/acceptance/features
# requires_gems: false
# requires_db: false
# - name: jest
# command: node_modules/.bin/jest --ci --json --coverage
# requires_gems: false
# requires_db: false
# - name: stylelint
# command: node_modules/.bin/stylelint "app/**/*.scss"
# requires_gems: false
# requires_db: false
name: ${{ matrix.name }}
services:
postgres:
image: postgres:11.6
env:
POSTGRES_USER: ${{ env.DB_USERNAME }}
POSTGRES_DB: ${{ env.DB_NAME }}
POSTGRES_PASSWORD: ${{ env.DB_PASSWORD }}
POSTGRES_HOST_AUTH_METHOD: ${{ env.POSTGRES_HOST_AUTH_METHOD }}
ports:
- 5432:5432
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Setup Ruby
uses: ruby/setup-ruby@v1
if: ${{ matrix.requires_gems }}
- name: Ruby gem cache
uses: actions/cache@v2
with:
path: vendor/bundle
key: ${{ runner.os }}-gems-${{ hashFiles('**/Gemfile.lock') }}
restore-keys: ${{ runner.os }}-gems-
if: ${{ matrix.requires_gems }}
- name: Install gems
run: |
bundle config path vendor/bundle
bundle install --jobs 4 --retry 3 --without production
if: ${{ matrix.requires_gems }}
- name: Setup test database
run: bin/rails db:create db:migrate
if: ${{ matrix.requires_db }}
- name: Run ${{ matrix.name }}
run: ${{ matrix.command }}
GitHub Actions is a feature that enables developers to automate their software workflows. With 2000 free minutes per month, developers can build, test, and deploy code automatically, integrating this functionality directly into their GitHub repositories. The automation includes critical processes like unit testing and deployment, which helps streamline continuous integration and continuous delivery (CI/CD) efforts.
GitHub Actions is particularly useful in ensuring that code remains functional and bug-free by running tests on every push or pull request. This saves developers time by allowing them to focus on writing code without worrying about manual deployment and testing.
To set up GitHub Actions for automated RSpec testing, the process starts by creating a folder structure within the repository: .github/workflows. Within this structure, a YAML file defines the specific actions that will run. The YAML file specifies when the actions should be triggered, typically on push or pull request events, and identifies the folder containing the code to be tested.
In the YAML configuration, developers define the operating environment for the tests, usually specifying the latest version of Ubuntu. The environment includes services like PostgreSQL, Redis, and Elasticsearch to mimic the actual production setup. These services are essential for ensuring the tests run accurately, particularly when dealing with databases and other back-end systems.
The YAML file includes commands to install dependencies like Ruby and PostgreSQL. Ruby for Rails projects, and PostgreSQL is necessary for running the database used in testing. After setting up these dependencies, the YAML script installs the necessary Ruby gems through bundle install. This ensures that all the libraries and packages needed for the project are available in the testing environment.
Once the environment is prepared, the script sets up the database by creating it and loading the schema. This step aligns the test environment's database structure with the codebase, ensuring that the tests run against a fully functional database setup.
The final step in the process is running the RSpec suite. RSpec is a popular testing framework for Ruby that allows developers to write tests that validate the correctness of their code. By running the tests automatically on every code push, developers can ensure that new changes do not break existing functionality. This automatic testing saves time and effort by catching bugs early in the development process, reducing the risk of errors making it to production.
One of the key advantages of this setup is that it eliminates the need for manual testing or deployment. The tests run automatically in the background whenever code is pushed to the repository. Developers can work with the assurance that their code is being tested, and once all tests pass, the code is ready for deployment. This process simplifies the workflow and ensures a more reliable, automated approach to testing and deployment.
GitHub Actions provides a free machine for this automation, significantly reducing infrastructure costs for teams. The machine is only activated when code is pushed or a pull request is created, allowing the team to use resources efficiently. Once the tests are completed, the machine can shut down, saving valuable computing time and resources.
Overall, automating testing with GitHub Actions enhances productivity and reduces errors in the development process. By setting up a continuous testing system with RSpec and integrating it into GitHub, developers can streamline their workflows, allowing them to focus on writing quality code. This approach ensures that the codebase remains stable, and developers can deploy updates with confidence, knowing that their tests will catch any potential issues early.
So this is how my github action looks like:
# .github/workflows/rails-tests.yml
env:
DB_NAME: talent_test
DB_USERNAME: postgres
DB_PASSWORD: postgres
DB_HOST: localhost
POSTGRES_HOST_AUTH_METHOD: trust
RAILS_ENV: test
name: Rails Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
strategy:
fail-fast: false
matrix:
include:
# - name: traceroute
# command: bin/rails traceroute
# requires_gems: true
# requires_db: false
# - name: brakeman
# command: bundle exec brakeman
# requires_gems: true
# requires_db: false
# - name: database_consistency
# command: bundle exec database_consistency
# requires_gems: true
# requires_db: true
# - name: erblint
# command: bundle exec erblint --lint-all
# requires_gems: true
# requires_db: false
- name: rspec_controllers
command: bundle exec rspec spec/controllers
requires_gems: true
requires_db: true
- name: rspec_helpers
command: bundle exec rspec spec/helpers spec/helpers
requires_gems: true
requires_db: true
- name: rspec_models
command: bundle exec rspec spec/mailers spec/models
requires_gems: true
requires_db: true
# - name: rspec_requests
# command: bundle exec rspec spec/requests
# requires_gems: true
# requires_db: true
# - name: rubocop
# command: bundle exec rubocop --parallel
# requires_gems: true
# requires_db: false
# - name: eslint
# command: node_modules/.bin/eslint --ext .js --ext .jsx --ext .json app/javascript
# requires_gems: false
# requires_db: false
# - name: gherkin-lint
# command: node_modules/.bin/gherkin-lint spec/acceptance/features
# requires_gems: false
# requires_db: false
# - name: jest
# command: node_modules/.bin/jest --ci --json --coverage
# requires_gems: false
# requires_db: false
# - name: stylelint
# command: node_modules/.bin/stylelint "app/**/*.scss"
# requires_gems: false
# requires_db: false
name: ${{ matrix.name }}
services:
postgres:
image: postgres:11.6
env:
POSTGRES_USER: ${{ env.DB_USERNAME }}
POSTGRES_DB: ${{ env.DB_NAME }}
POSTGRES_PASSWORD: ${{ env.DB_PASSWORD }}
POSTGRES_HOST_AUTH_METHOD: ${{ env.POSTGRES_HOST_AUTH_METHOD }}
ports:
- 5432:5432
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Setup Ruby
uses: ruby/setup-ruby@v1
if: ${{ matrix.requires_gems }}
- name: Ruby gem cache
uses: actions/cache@v2
with:
path: vendor/bundle
key: ${{ runner.os }}-gems-${{ hashFiles('**/Gemfile.lock') }}
restore-keys: ${{ runner.os }}-gems-
if: ${{ matrix.requires_gems }}
- name: Install gems
run: |
bundle config path vendor/bundle
bundle install --jobs 4 --retry 3 --without production
if: ${{ matrix.requires_gems }}
- name: Setup test database
run: bin/rails db:create db:migrate
if: ${{ matrix.requires_db }}
- name: Run ${{ matrix.name }}
run: ${{ matrix.command }}
GitHub Actions is a feature that enables developers to automate their software workflows. With 2000 free minutes per month, developers can build, test, and deploy code automatically, integrating this functionality directly into their GitHub repositories. The automation includes critical processes like unit testing and deployment, which helps streamline continuous integration and continuous delivery (CI/CD) efforts.
GitHub Actions is particularly useful in ensuring that code remains functional and bug-free by running tests on every push or pull request. This saves developers time by allowing them to focus on writing code without worrying about manual deployment and testing.
To set up GitHub Actions for automated RSpec testing, the process starts by creating a folder structure within the repository: .github/workflows. Within this structure, a YAML file defines the specific actions that will run. The YAML file specifies when the actions should be triggered, typically on push or pull request events, and identifies the folder containing the code to be tested.
In the YAML configuration, developers define the operating environment for the tests, usually specifying the latest version of Ubuntu. The environment includes services like PostgreSQL, Redis, and Elasticsearch to mimic the actual production setup. These services are essential for ensuring the tests run accurately, particularly when dealing with databases and other back-end systems.
The YAML file includes commands to install dependencies like Ruby and PostgreSQL. Ruby for Rails projects, and PostgreSQL is necessary for running the database used in testing. After setting up these dependencies, the YAML script installs the necessary Ruby gems through bundle install. This ensures that all the libraries and packages needed for the project are available in the testing environment.
Once the environment is prepared, the script sets up the database by creating it and loading the schema. This step aligns the test environment's database structure with the codebase, ensuring that the tests run against a fully functional database setup.
The final step in the process is running the RSpec suite. RSpec is a popular testing framework for Ruby that allows developers to write tests that validate the correctness of their code. By running the tests automatically on every code push, developers can ensure that new changes do not break existing functionality. This automatic testing saves time and effort by catching bugs early in the development process, reducing the risk of errors making it to production.
One of the key advantages of this setup is that it eliminates the need for manual testing or deployment. The tests run automatically in the background whenever code is pushed to the repository. Developers can work with the assurance that their code is being tested, and once all tests pass, the code is ready for deployment. This process simplifies the workflow and ensures a more reliable, automated approach to testing and deployment.
GitHub Actions provides a free machine for this automation, significantly reducing infrastructure costs for teams. The machine is only activated when code is pushed or a pull request is created, allowing the team to use resources efficiently. Once the tests are completed, the machine can shut down, saving valuable computing time and resources.
Overall, automating testing with GitHub Actions enhances productivity and reduces errors in the development process. By setting up a continuous testing system with RSpec and integrating it into GitHub, developers can streamline their workflows, allowing them to focus on writing quality code. This approach ensures that the codebase remains stable, and developers can deploy updates with confidence, knowing that their tests will catch any potential issues early.
So this is how my github action looks like:
# .github/workflows/rails-tests.yml
env:
DB_NAME: talent_test
DB_USERNAME: postgres
DB_PASSWORD: postgres
DB_HOST: localhost
POSTGRES_HOST_AUTH_METHOD: trust
RAILS_ENV: test
name: Rails Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
strategy:
fail-fast: false
matrix:
include:
# - name: traceroute
# command: bin/rails traceroute
# requires_gems: true
# requires_db: false
# - name: brakeman
# command: bundle exec brakeman
# requires_gems: true
# requires_db: false
# - name: database_consistency
# command: bundle exec database_consistency
# requires_gems: true
# requires_db: true
# - name: erblint
# command: bundle exec erblint --lint-all
# requires_gems: true
# requires_db: false
- name: rspec_controllers
command: bundle exec rspec spec/controllers
requires_gems: true
requires_db: true
- name: rspec_helpers
command: bundle exec rspec spec/helpers spec/helpers
requires_gems: true
requires_db: true
- name: rspec_models
command: bundle exec rspec spec/mailers spec/models
requires_gems: true
requires_db: true
# - name: rspec_requests
# command: bundle exec rspec spec/requests
# requires_gems: true
# requires_db: true
# - name: rubocop
# command: bundle exec rubocop --parallel
# requires_gems: true
# requires_db: false
# - name: eslint
# command: node_modules/.bin/eslint --ext .js --ext .jsx --ext .json app/javascript
# requires_gems: false
# requires_db: false
# - name: gherkin-lint
# command: node_modules/.bin/gherkin-lint spec/acceptance/features
# requires_gems: false
# requires_db: false
# - name: jest
# command: node_modules/.bin/jest --ci --json --coverage
# requires_gems: false
# requires_db: false
# - name: stylelint
# command: node_modules/.bin/stylelint "app/**/*.scss"
# requires_gems: false
# requires_db: false
name: ${{ matrix.name }}
services:
postgres:
image: postgres:11.6
env:
POSTGRES_USER: ${{ env.DB_USERNAME }}
POSTGRES_DB: ${{ env.DB_NAME }}
POSTGRES_PASSWORD: ${{ env.DB_PASSWORD }}
POSTGRES_HOST_AUTH_METHOD: ${{ env.POSTGRES_HOST_AUTH_METHOD }}
ports:
- 5432:5432
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Setup Ruby
uses: ruby/setup-ruby@v1
if: ${{ matrix.requires_gems }}
- name: Ruby gem cache
uses: actions/cache@v2
with:
path: vendor/bundle
key: ${{ runner.os }}-gems-${{ hashFiles('**/Gemfile.lock') }}
restore-keys: ${{ runner.os }}-gems-
if: ${{ matrix.requires_gems }}
- name: Install gems
run: |
bundle config path vendor/bundle
bundle install --jobs 4 --retry 3 --without production
if: ${{ matrix.requires_gems }}
- name: Setup test database
run: bin/rails db:create db:migrate
if: ${{ matrix.requires_db }}
- name: Run ${{ matrix.name }}
run: ${{ matrix.command }}
GitHub Actions is a feature that enables developers to automate their software workflows. With 2000 free minutes per month, developers can build, test, and deploy code automatically, integrating this functionality directly into their GitHub repositories. The automation includes critical processes like unit testing and deployment, which helps streamline continuous integration and continuous delivery (CI/CD) efforts.
GitHub Actions is particularly useful in ensuring that code remains functional and bug-free by running tests on every push or pull request. This saves developers time by allowing them to focus on writing code without worrying about manual deployment and testing.
To set up GitHub Actions for automated RSpec testing, the process starts by creating a folder structure within the repository: .github/workflows. Within this structure, a YAML file defines the specific actions that will run. The YAML file specifies when the actions should be triggered, typically on push or pull request events, and identifies the folder containing the code to be tested.
In the YAML configuration, developers define the operating environment for the tests, usually specifying the latest version of Ubuntu. The environment includes services like PostgreSQL, Redis, and Elasticsearch to mimic the actual production setup. These services are essential for ensuring the tests run accurately, particularly when dealing with databases and other back-end systems.
The YAML file includes commands to install dependencies like Ruby and PostgreSQL. Ruby for Rails projects, and PostgreSQL is necessary for running the database used in testing. After setting up these dependencies, the YAML script installs the necessary Ruby gems through bundle install. This ensures that all the libraries and packages needed for the project are available in the testing environment.
Once the environment is prepared, the script sets up the database by creating it and loading the schema. This step aligns the test environment's database structure with the codebase, ensuring that the tests run against a fully functional database setup.
The final step in the process is running the RSpec suite. RSpec is a popular testing framework for Ruby that allows developers to write tests that validate the correctness of their code. By running the tests automatically on every code push, developers can ensure that new changes do not break existing functionality. This automatic testing saves time and effort by catching bugs early in the development process, reducing the risk of errors making it to production.
One of the key advantages of this setup is that it eliminates the need for manual testing or deployment. The tests run automatically in the background whenever code is pushed to the repository. Developers can work with the assurance that their code is being tested, and once all tests pass, the code is ready for deployment. This process simplifies the workflow and ensures a more reliable, automated approach to testing and deployment.
GitHub Actions provides a free machine for this automation, significantly reducing infrastructure costs for teams. The machine is only activated when code is pushed or a pull request is created, allowing the team to use resources efficiently. Once the tests are completed, the machine can shut down, saving valuable computing time and resources.
Overall, automating testing with GitHub Actions enhances productivity and reduces errors in the development process. By setting up a continuous testing system with RSpec and integrating it into GitHub, developers can streamline their workflows, allowing them to focus on writing quality code. This approach ensures that the codebase remains stable, and developers can deploy updates with confidence, knowing that their tests will catch any potential issues early.
So this is how my github action looks like:
# .github/workflows/rails-tests.yml
env:
DB_NAME: talent_test
DB_USERNAME: postgres
DB_PASSWORD: postgres
DB_HOST: localhost
POSTGRES_HOST_AUTH_METHOD: trust
RAILS_ENV: test
name: Rails Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
strategy:
fail-fast: false
matrix:
include:
# - name: traceroute
# command: bin/rails traceroute
# requires_gems: true
# requires_db: false
# - name: brakeman
# command: bundle exec brakeman
# requires_gems: true
# requires_db: false
# - name: database_consistency
# command: bundle exec database_consistency
# requires_gems: true
# requires_db: true
# - name: erblint
# command: bundle exec erblint --lint-all
# requires_gems: true
# requires_db: false
- name: rspec_controllers
command: bundle exec rspec spec/controllers
requires_gems: true
requires_db: true
- name: rspec_helpers
command: bundle exec rspec spec/helpers spec/helpers
requires_gems: true
requires_db: true
- name: rspec_models
command: bundle exec rspec spec/mailers spec/models
requires_gems: true
requires_db: true
# - name: rspec_requests
# command: bundle exec rspec spec/requests
# requires_gems: true
# requires_db: true
# - name: rubocop
# command: bundle exec rubocop --parallel
# requires_gems: true
# requires_db: false
# - name: eslint
# command: node_modules/.bin/eslint --ext .js --ext .jsx --ext .json app/javascript
# requires_gems: false
# requires_db: false
# - name: gherkin-lint
# command: node_modules/.bin/gherkin-lint spec/acceptance/features
# requires_gems: false
# requires_db: false
# - name: jest
# command: node_modules/.bin/jest --ci --json --coverage
# requires_gems: false
# requires_db: false
# - name: stylelint
# command: node_modules/.bin/stylelint "app/**/*.scss"
# requires_gems: false
# requires_db: false
name: ${{ matrix.name }}
services:
postgres:
image: postgres:11.6
env:
POSTGRES_USER: ${{ env.DB_USERNAME }}
POSTGRES_DB: ${{ env.DB_NAME }}
POSTGRES_PASSWORD: ${{ env.DB_PASSWORD }}
POSTGRES_HOST_AUTH_METHOD: ${{ env.POSTGRES_HOST_AUTH_METHOD }}
ports:
- 5432:5432
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Setup Ruby
uses: ruby/setup-ruby@v1
if: ${{ matrix.requires_gems }}
- name: Ruby gem cache
uses: actions/cache@v2
with:
path: vendor/bundle
key: ${{ runner.os }}-gems-${{ hashFiles('**/Gemfile.lock') }}
restore-keys: ${{ runner.os }}-gems-
if: ${{ matrix.requires_gems }}
- name: Install gems
run: |
bundle config path vendor/bundle
bundle install --jobs 4 --retry 3 --without production
if: ${{ matrix.requires_gems }}
- name: Setup test database
run: bin/rails db:create db:migrate
if: ${{ matrix.requires_db }}
- name: Run ${{ matrix.name }}
run: ${{ matrix.command }}
Guadalajara
Werkshop - Av. Acueducto 6050, Lomas del bosque, Plaza Acueducto. 45116,
Zapopan, Jalisco. México.
Texas
5700 Granite Parkway, Suite 200, Plano, Texas 75024.
© Density Labs. All Right reserved. Privacy policy and Terms of Use.
Guadalajara
Werkshop - Av. Acueducto 6050, Lomas del bosque, Plaza Acueducto. 45116,
Zapopan, Jalisco. México.
Texas
5700 Granite Parkway, Suite 200, Plano, Texas 75024.
© Density Labs. All Right reserved. Privacy policy and Terms of Use.
Guadalajara
Werkshop - Av. Acueducto 6050, Lomas del bosque, Plaza Acueducto. 45116,
Zapopan, Jalisco. México.
Texas
5700 Granite Parkway, Suite 200, Plano, Texas 75024.
© Density Labs. All Right reserved. Privacy policy and Terms of Use.